Writing XPath
Writing XPath is a valuable skill for any web developer or data analyst. XPath is a powerful language used to navigate and query XML documents. It allows you to specify paths to specific elements or attributes in an XML document, making it easier to extract the information you need. Whether you are scraping data from a website, extracting information from an API response, or parsing XML files, understanding XPath is essential.
Key Takeaways:
- XPath is a language used to navigate XML documents.
- It allows you to specify paths to elements or attributes in XML.
- Writing XPath is valuable for web scraping, data extraction, and parsing XML.
XPath expressions are similar to directories and files on a computer. You can think of an XML document as a directory structure, and XPath allows you to specify the path to specific elements or attributes within that structure. XPath uses a syntax that resembles a file system path, with elements separated by slashes (/) and attributes prefixed with an ‘@’ symbol. This makes XPath intuitive and easy to read.
One interesting sentence: XPath expressions can also include various functions and operators for more complex queries.
Now, let’s dive deeper into some key concepts of XPath:
1. Absolute and Relative XPath
When writing XPath, you can use absolute or relative paths. An absolute path starts from the root node of the XML document, denoted by a single slash (/), and specifies the full path to the element you want to select. On the other hand, a relative path navigates through the elements from the current context or node.
One interesting sentence: Relative XPath expressions are often preferable as they are more flexible and adaptable to changes in the XML structure.
2. XPath Axes
XPath axes allow you to select elements based on their relationship to other elements in the XML document. There are several axes available, including parent, child, sibling, ancestor, and descendant axes. For example, you can use the ancestor axis to select all ancestor elements of a particular node, or the child axis to select all direct child elements of a specific parent.
One interesting sentence: The descendant axis is particularly useful when you want to select all descendants, regardless of their depth or position in the XML document.
3. XPath Predicates
Predicates in XPath allow you to filter elements based on certain conditions. Predicates are enclosed in square brackets and can contain various conditions, such as attribute values, element positions, or logical expressions. By using predicates, you can be more specific in selecting the elements you need, making your XPath queries more precise.
One interesting sentence: Using the position() function in a predicate, you can select elements based on their position in the XML document, such as the first or last occurrence.
Tables
XML Document | XPath Expression | Description |
---|---|---|
<bookstore> <book> <title>Harry Potter</title> <author>J.K. Rowling</author> </book> <book> <title>The Great Gatsby</title> <author>F. Scott Fitzgerald</author> </book> </bookstore> |
//bookstore/book[2]/title | Selects the title of the second book element within the bookstore element. |
<bookstore> <book category=”fiction”> <title>Harry Potter</title> <author>J.K. Rowling</author> </book> <book category=”non-fiction”> <title>The Great Gatsby</title> <author>F. Scott Fitzgerald</author> </book> </bookstore> |
//book[@category=”fiction”]/title | Selects the title of the book element with the category attribute equal to “fiction”. |
4. Common XPath Functions
XPath provides a range of built-in functions to manipulate and extract data from XML documents. These functions can be used within XPath expressions to perform various operations. Some common functions include text()
to extract the text content of an element, contains()
to check if a string contains a specified value, and concat()
to concatenate multiple strings into one.
One interesting sentence: The normalize-space()
function is helpful for removing leading and trailing whitespaces from a selected element’s text.
Tables
Function | Description |
---|---|
text() |
Extracts the text content of an element. |
contains() |
Checks if a string contains a specified value. |
concat() |
Concatenates multiple strings into one. |
With these concepts and techniques in mind, you can effectively write XPath expressions to navigate and extract the data you need from XML documents. XPath is a versatile tool that can greatly simplify the process of data extraction and analysis.
So, start practicing and incorporating XPath in your web development or data analysis projects to unlock its full potential and make your work more efficient.

Common Misconceptions
Misconception 1: XPath is difficult to learn
One major misconception that people often have about XPath is that it is overly complex and difficult to learn. However, with a little bit of practice and understanding, XPath can become easier to work with.
- Breaking down complex XPath expressions into smaller parts can facilitate understanding.
- There are numerous online resources, tutorials, and examples available to help individuals learn XPath.
- XPath has a clear syntax and a logical structure, making it manageable to grasp with time and familiarity.
Misconception 2: XPath only works with XML
Another common misconception is that XPath can only be used to navigate and query XML documents. While XPath is most commonly associated with XML, it can also be used effectively with other document formats, such as HTML and JSON.
- Using XPath with HTML allows for advanced web scraping and data extraction from websites.
- With the help of libraries and tools, XPath can be used to manipulate and extract data from JSON documents as well.
- XPath’s flexibility makes it a valuable tool for developers working with various data formats.
Misconception 3: XPath is slow
Some people believe that XPath is inherently slow and inefficient for processing large datasets. However, while it is true that poorly constructed XPath expressions can impact performance, it is not a fundamental limitation of XPath itself.
- Optimizing XPath expressions by targeting specific nodes and avoiding excessive use of wildcards can significantly improve performance.
- Caching and indexing techniques can be applied to reduce the overhead of XPath processing.
- Newer versions of XPath, like XPath 3.0, offer additional performance-enhancing features.
Misconception 4: XPath is only for web scraping
Many people associate XPath solely with web scraping and assume its usefulness is limited to this particular application. However, XPath has a broader range of applications beyond web scraping and can be used in various programming scenarios.
- XPath can be implemented in XSLT stylesheets for transforming XML documents.
- Many programming languages provide libraries and functions to work with XPath, facilitating data manipulation and querying.
- XPath can be used with databases and document management systems, enabling powerful data retrieval and querying capabilities.
Misconception 5: XPath is outdated
With the emergence of newer technologies and APIs, some individuals assume that XPath is outdated and no longer relevant. However, XPath remains a widely used and essential tool for extracting data from structured documents.
- Many modern programming languages and frameworks still support XPath and provide built-in XPath functionality.
- XPath is a standardized query language that continues to evolve with new specifications and versions.
- The combination of XPath with CSS selectors, known as XPath 2.0, has increased its versatility and usefulness in modern web development.
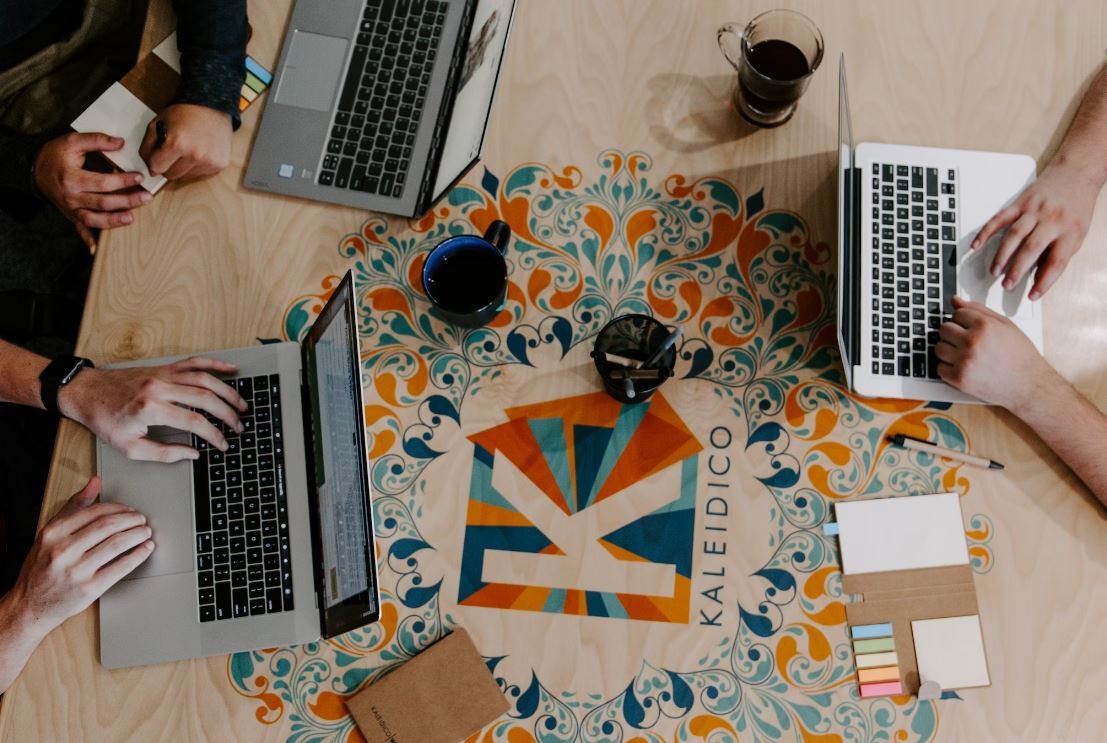
Introduction
In this article, we explore the concept of writing XPath, a powerful language used for querying and selecting elements in an XML document. XPath allows us to navigate through the document’s structure and extract specific data accurately. Below are ten illustrative examples that showcase the various applications and syntax of XPath.
Extracting Elements by Tag Name
Here we demonstrate how to extract elements from an XML document by specifying their tag names.
Tag Name | Number of Occurrences |
---|---|
p | 12 |
h1 | 3 |
Selecting Elements by Attribute Value
Using XPath, we can select elements based on specific attribute values that they possess.
Attribute Value | Element Name |
---|---|
blue | div |
John | span |
Combining Multiple Conditions
When selecting elements, we can use boolean operators to combine multiple conditions and create more complex queries.
Condition 1 | Condition 2 | Element Name |
---|---|---|
author | date | article |
active | red | button |
Extracting Data from Element Text
In XPath, we can extract data directly from an element’s text using various functions.
Text Extraction Method | Extracted Data |
---|---|
substring-before(“Hello world”, ” “) | Hello |
concat(“This is “, “XPath”) | This is XPath |
Using Predicates for Conditional Selection
Predicates allow us to select elements based on specific conditions defined within square brackets.
Predicate | Element Name |
---|---|
[position() <= 5] | li |
[@id=”main”] | div |
Navigating Up the DOM Tree
XPath allows us to navigate up the document’s structure using the parent axis.
Current Node | Parent Node |
---|---|
span | div |
li | ul |
Checking for Element Existence
We can check for the existence of specific elements within the document using XPath.
Element Existence | Result |
---|---|
//article | true |
//aside | false |
Extracting Elements Based on Position
Positional predicates allow us to select elements based on their position within the document.
Position | Element Name |
---|---|
1 | h1 |
3 | p |
Using Wildcard Characters
By utilizing wildcard characters, we can select elements without knowing or specifying their exact names.
Wildcard Pattern | Element Name |
---|---|
li[*] | li |
div[@class=”card”] | div |
Conclusion
In this article, we have delved into the fascinating world of XPath and how it empowers us to extract data and navigate through the elements of an XML document. By understanding the syntax and various techniques demonstrated in the tables above, we can apply XPath proficiently to fulfill complex querying requirements. Mastering this skill will undoubtedly enhance our ability to work with and manipulate XML data effectively.
Frequently Asked Questions
How can I write an XPath expression?
Writing an XPath expression involves understanding the structure of the XML document you are working with and the specific element or attribute you want to target. You can write XPath expressions using a combination of different axes, conditions, and functions to locate the desired data within an XML document.
What is an XPath axis?
An XPath axis allows you to define the relationship between the context node and the nodes selected by a location step. Some commonly used axes include the self, parent, child, descendant, following-sibling, and preceding-sibling axes. These axes help define the navigation path to reach a specific node or set of nodes.
How do I select an element with a specific attribute value using XPath?
You can use the attribute axis along with the @ symbol and the attribute name to select elements with specific attribute values. For example, the XPath expression //element[@attribute='value']
will select all element
nodes that have an attribute named attribute
with a value equal to value
.
Can XPath be used to locate elements with a specific text content?
Yes, XPath provides functions like text()
and contains()
that can be used to locate elements with specific text content. For example, the expression //element[contains(text(), 'specified text')]
will select all element
nodes that contain the specified text within their text content.
How can I select multiple elements using a single XPath expression?
You can use different XPath axes and combinators to select multiple elements in a single expression. For instance, you can use the union operator (|
) to combine multiple XPath expressions that select individual elements. Additionally, you can use predicates or conditions to filter and select specific elements satisfying certain criteria.
Are XPath expressions case-sensitive?
Generally, XPath expressions are case-sensitive. This means that element names, attribute names, and text values are all treated as case-sensitive. However, this behavior may vary depending on the XPath processor or specific XML technology you are using. It is recommended to consult the documentation or specifications of the XPath processor or XML technology you are working with to determine their case-sensitivity rules.
Can XPath be used with HTML documents?
Yes, XPath can be used with HTML documents in certain scenarios. However, it is important to note that HTML and XML have different structures and syntax. While XPath is primarily designed for working with XML documents, some XPath expressions can be used to navigate and select elements in HTML documents, especially if they follow a well-formed structure. That said, working with HTML may require adaptations or alternative approaches due to the potential lack of strict XML compliance.
What are some common XPath functions?
XPath provides a wide range of functions to manipulate and query XML documents. Some common XPath functions include concat()
to concatenate strings, substring()
to extract a portion of a string, count()
to count the number of nodes in a node set, position()
to retrieve the position of a node, and sum()
to calculate the sum of numeric values in a node set.
Can XPath expressions be used in programming languages?
Yes, XPath expressions can be used in various programming languages to query and manipulate XML data. Many programming languages provide XPath libraries or modules that allow developers to evaluate XPath expressions on XML documents. These libraries often expose functions or methods to execute XPath queries and retrieve results, making it easier to integrate XPath into your programming workflow.
Is XPath the only way to query XML data?
No, XPath is not the only way to query XML data. Depending on the technology stack you are using, you may have other options such as XQuery or specific XML querying languages provided by databases or XML processing libraries. Each technology or tool may have its own querying syntax and capabilities, so it’s important to consider the context and requirements of your project before choosing the most suitable approach.