Writing x86 Assembly
Assembly language is a low-level programming language that allows direct control over the computer’s hardware, providing efficiency and fine-grained control. x86 is one of the most widely used assembly language architectures and is compatible with many popular operating systems and processors.
Key Takeaways
- Assembly language is a low-level programming language.
- x86 is a widely used assembly language architecture.
- x86 assembly provides efficiency and fine-grained control over hardware.
**Writing x86 assembly code requires an understanding of the computer’s architecture and instruction set**. Each x86 instruction corresponds to a specific operation performed by the computer’s CPU. By writing assembly code, programmers gain control over the computer’s resources at a low level, allowing them to optimize performance and interact with hardware directly. Assembly code is written using mnemonics, which are human-readable representations of machine instructions.
In x86 assembly, **registers play a crucial role**. Registers are small, fast memory locations within the CPU that can hold data or addresses. They act as temporary storage for the CPU during computations. x86 assembly has eight general-purpose registers, each with a specific purpose. They can be used to manipulate data, perform arithmetic operations, and control program flow.
**Memory access is an essential aspect of assembly programming**. x86 architecture provides various addressing modes, allowing efficient access to memory locations. Direct addressing, register indirect addressing, and base + index addressing are some of the commonly used addressing modes in x86 assembly. Having different addressing modes allows flexibility when accessing and manipulating data in memory.
Data Representation
In x86 assembly, data is represented using different data types, such as bytes, words, and double words. These data types determine how much memory is allocated to store the data. For example, a byte represents 8 bits of data, whereas a word represents 16 bits and a double word represents 32 bits.
Instructions and Directives
**x86 assembly instructions** are the set of commands that the CPU understands and executes. These instructions perform operations such as arithmetic, logical, data transfer, and control flow. Each instruction has a specific opcode and can also take operands to determine the exact behavior of the instruction.
**Directives** are special instructions that provide additional information to the assembler, helping with the assembly process. They are not executed at runtime but are instructions for the assembler itself. Directives can define constants, allocate memory, specify the start of the program, and more. They play an important role in organizing and structuring assembly code.
Tables
Register | Description |
---|---|
EAX | Accumulator register |
EBX | Base register |
ECX | Counter register |
EDX | Data register |
ESI | Source index register |
EDI | Destination index register |
EBP | Base pointer register |
ESP | Stack pointer register |
Mode | Description |
---|---|
Direct Addressing | Access data directly using a memory address |
Register Indirect Addressing | Access data indirectly using a register that holds the memory address |
Base + Index Addressing | Access data using a combination of a base register and an index register |
Type | Size (bits) |
---|---|
Byte | 8 |
Word | 16 |
Double Word | 32 |
**Writing x86 assembly** can be challenging, but it provides a deep understanding of how computer systems work at a fundamental level, offering fine control over performance and hardware resources. By utilizing the x86 instruction set, handling registers efficiently, and understanding the various addressing modes, programmers can optimize code execution and build efficient systems.
Whether you’re working on system-level programming, reverse engineering, or low-level optimization, **learning x86 assembly opens up a world of possibilities** and greatly expands your understanding of computer architecture.
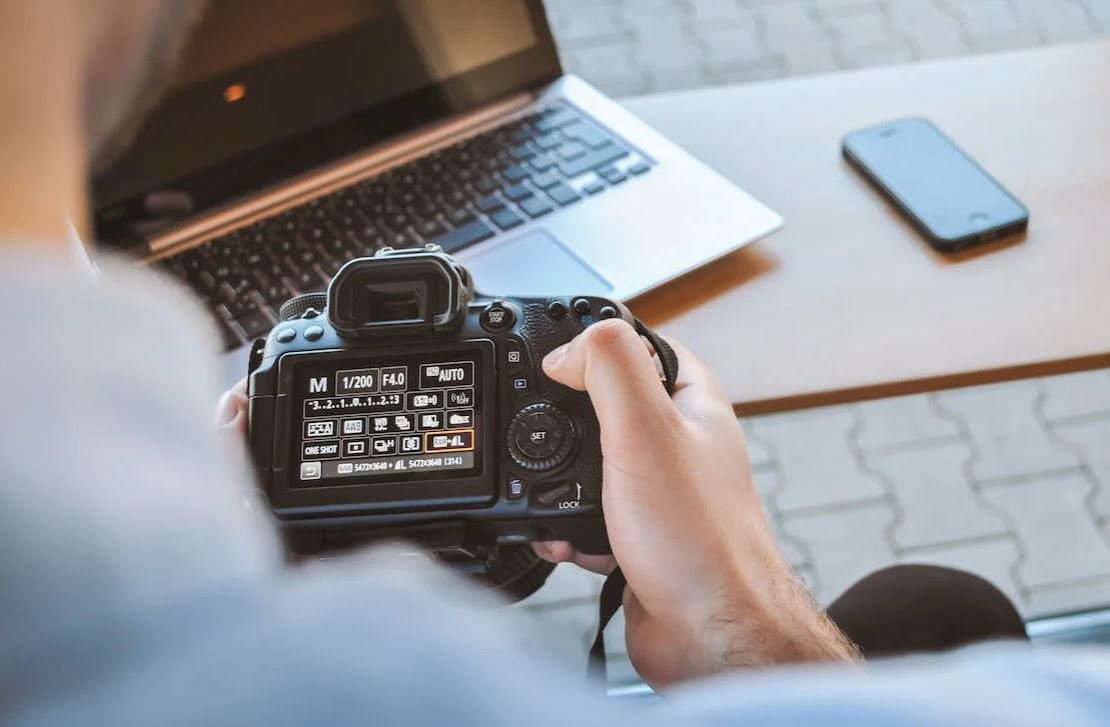
Common Misconceptions
Paragraph 1
One common misconception people have about writing x86 Assembly is that it is excessively complex and difficult to learn. However, with proper guidance and practice, anyone can grasp the basics of assembly programming.
- Assembly language is based on simple instructions
- A good understanding of computer architecture is helpful
- Many online resources and tutorials are available to assist in learning
Paragraph 2
Another misconception is that assembly language is obsolete and no longer relevant in modern computing. While it is true that high-level languages like C and Java are more commonly used, understanding assembly can provide valuable insights into low-level programming and optimization.
- Assembly is used in operating system development
- Efficient code optimization often requires knowledge of assembly
- Understanding assembly improves debugging skills
Paragraph 3
It is often incorrectly assumed that writing code in assembly language is only necessary for specific system tasks or highly specialized applications. However, there are various scenarios where assembly programming can be beneficial, such as embedded systems programming, real-time applications, and performance-critical tasks.
- Embedded systems rely on assembly for hardware interaction
- Certain real-time applications require precise control over processes
- High-performance computing can greatly benefit from assembly optimization
Paragraph 4
Many people believe that assembly language is too time-consuming compared to high-level languages. While it is true that writing assembly requires more effort, it can often result in highly optimized and efficient code that performs better than equivalent code written in a high-level language.
- Assembly provides fine-grained control over hardware resources
- Optimized assembly code can outperform equivalent high-level code
- Profiling tools can aid in identifying performance bottlenecks
Paragraph 5
Finally, some individuals believe that learning assembly language is unnecessary for a career in software development. While it may not be a requirement for every software development role, understanding assembly can enhance one’s comprehension of computer systems and enable deeper problem-solving skills.
- Assembly knowledge helps in reverse engineering
- Understanding assembly improves debugging capabilities
- Assembly programming can lead to a deeper understanding of high-level languages
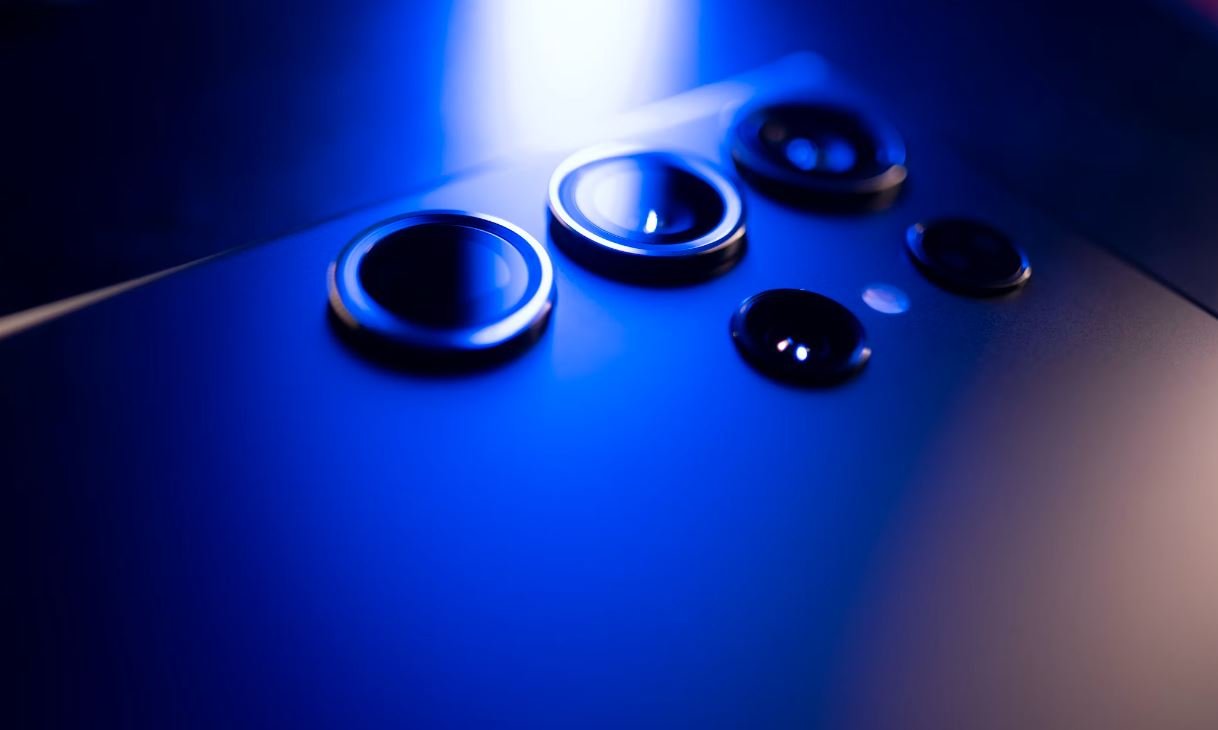
Introduction
Writing x86 Assembly is a fascinating skill that allows programmers to have low-level control and optimize code. In this article, we will explore various aspects of x86 Assembly programming through 10 engaging tables. Each table presents important data or points to enrich our understanding of this topic. So, let’s dive in!
A Brief History of Assembly Languages
Before we delve into the specifics of x86 Assembly, let’s take a moment to appreciate the rich history of assembly languages. Assembly languages have been evolving since the early days of computers, providing a bridge between machine code and high-level languages. The following table showcases some key milestones in assembly language development:
Year | Assembly Language | Significance |
---|---|---|
1949 | BINAC Assembly | First assembly language used on the BINAC computer. |
1951 | Short Code | Considered the first assembly language, it represented instructions using four decimal digits. |
1952 | SOAP | Symbolic Optimal Assembly Program, introduced macro facilities. |
1955 | FLOW-MATIC | Developed by Grace Hopper, it was the foundation for COBOL. |
Characteristics of x86 Assembly
The x86 Assembly language, widely used in Intel-compatible computers, offers unique characteristics that make it powerful and versatile. The following table highlights some notable aspects of x86 Assembly:
Characteristic | Description |
---|---|
Low-Level Language | Provides direct control over hardware resources and enables precise optimizations. |
Processor-Specific | Instructions are tailored to specific CPUs, such as Intel or AMD. |
Register-Based | Utilizes various registers for efficient data manipulation and storage. |
Compact Code | Instructions are shorter and denser compared to high-level languages. |
Assembly vs. High-Level Languages
Understanding the advantages and differences between assembly language and high-level languages is crucial for developers. The following table highlights key distinctions:
Aspect | Assembly Language | High-Level Language |
---|---|---|
Abstraction Level | Low level | High level |
Control | High control over hardware | Abstracted hardware access |
Readability | Less readable due to complex syntax | More readable with intuitive syntax |
Optimization | Fine-grained optimization | Compiler-driven optimization |
Benefits of Learning Assembly
Learning assembly language can be highly rewarding for programmers. It provides valuable insights into how computers work at a fundamental level and offers several benefits:
Benefit | Description |
---|---|
Performance Optimization | Ability to optimize critical sections of code for maximum speed and efficiency. |
Debugging Power | Detailed understanding of program flow and memory management. |
Portability | Assembly code can be used across various x86-based platforms. |
Reverse Engineering | Enables analysis of compiled software and understanding of its inner workings. |
Popular Assemblers
Various assemblers are available to write x86 Assembly code. Below, we present some widely used assemblers and their characteristics:
Assembler | Description |
---|---|
NASM | Netwide Assembler is highly portable and widely used for x86 and x86-64 Assembly. |
MASM | Microsoft Macro Assembler, often used for Windows-based development. |
Gas | GNU Assembler, commonly used in Linux and Unix environments. |
FASM | Flat Assembler, known for its flat memory model. |
x86 Instructions by Category
The x86 Assembly language consists of various instruction categories, each serving a specific purpose. The following table showcases some important instruction categories:
Category | Description |
---|---|
Data Transfer | Moves data between memory and registers or registers and registers. |
Arithmetic | Perform operations like addition, subtraction, multiplication, and division. |
Control Flow | Alter the flow of program execution using conditions and loops. |
Bit Manipulation | Manipulate bits within registers, often used for data encoding. |
Example: Fibonacci Sequence in Assembly
Let’s see the power of x86 Assembly by examining an implementation of the Fibonacci sequence:
N | Fibonacci(N) |
---|---|
0 | 0 |
1 | 1 |
2 | 1 |
3 | 2 |
4 | 3 |
5 | 5 |
6 | 8 |
7 | 13 |
8 | 21 |
Conclusion
Mastering x86 Assembly is an intriguing journey that unlocks a deeper understanding of computer systems and provides unparalleled control over code optimization. Through this article, we have explored various aspects of x86 Assembly, including its history, characteristics, benefits, assemblers, instruction categories, and even an example implementation of the Fibonacci sequence. Armed with this knowledge, programmers can embrace the intricacies of assembly language and leverage its power to write efficient and high-performance software.
Frequently Asked Questions
Writing x86 Assembly
FAQs
-
What is x86 Assembly?
-
Why should I learn x86 Assembly?
-
How difficult is it to learn x86 Assembly?
-
What tools do I need to write x86 Assembly code?
-
Can I run x86 Assembly code on any computer?
-
Is x86 Assembly still relevant in modern computing?
-
Where can I find resources to learn x86 Assembly?
-
What are some popular use cases for x86 Assembly?
-
What are some common pitfalls in x86 Assembly programming?
-
Can x86 Assembly be used in web development?