Writing XML in C#
XML (eXtensible Markup Language) is a widely used language for representing structured data. In C#, writing XML is a straightforward process that allows you to create, manipulate, and save XML documents. In this article, we will explore the basics of writing XML in C#, including creating XML elements, attributes, and nodes, as well as saving the XML data to a file.
Key Takeaways:
- XML is a language for representing structured data.
- C# provides a convenient way to write XML documents.
- C# allows you to create XML elements, attributes, and nodes.
- You can save XML data to a file using C#.
In C#, you can use the XmlDocument class from the System.Xml namespace to create and manipulate XML documents. To start, you need to create an instance of the XmlDocument class:
XmlDocument xmlDoc = new XmlDocument();
Once you have created an XmlDocument instance, you can start building the XML structure using various methods and properties:
- CreateElement: Creates a new XML element.
- CreateAttribute: Creates a new XML attribute.
- CreateTextNode: Creates a new text node.
- AppendChild: Adds a child node to an XML element.
After creating an XML element and setting its attributes and text content, you can add it to the XML document using the AppendChild method. Here’s an example that demonstrates how to create a simple XML document:
XmlDocument xmlDoc = new XmlDocument(); XmlNode root = xmlDoc.CreateElement("Root"); xmlDoc.AppendChild(root); XmlNode child = xmlDoc.CreateElement("Child"); XmlNode textNode = xmlDoc.CreateTextNode("Hello, World!"); child.AppendChild(textNode); root.AppendChild(child);
Using the XmlDocument class, you can easily build complex XML structures and set attributes or text content for each element.
Saving XML Data to a File
Once you have built an XML document in C#, you can save it to a file using the Save method. The Save method takes a filename as a parameter and writes the XML data to that file. Here’s an example:
xmlDoc.Save("path/to/file.xml");
You can also specify different options while saving the XML data, such as preserving whitespace or encoding. The Save method provides overloads that enable you to customize the saving process.
Saving XML data to a file is a simple process with the Save method, allowing you to store your XML documents for later use or sharing.
XML Parsing Performance Comparison
In addition to writing XML in C#, it’s essential to consider the performance implications of different approaches. Let’s compare the performance of two commonly used XML parsing methods:
Method | Pros | Cons |
---|---|---|
XmlTextWriter | Fast and efficient for writing XML. | Does not provide built-in support for reading XML. |
XmlDocument | Provides full support for reading and writing XML. | Can be slower and less memory-efficient for large XML documents. |
Considering the performance characteristics of different XML parsing methods helps ensure efficient handling of XML data in your C# applications.
Conclusion
Writing XML in C# is a powerful way to create and manipulate structured data. With the XmlDocument class, you can easily build complex XML structures and save XML documents to files. Consider the performance implications of different XML parsing methods to optimize your C# applications for handling XML data efficiently.
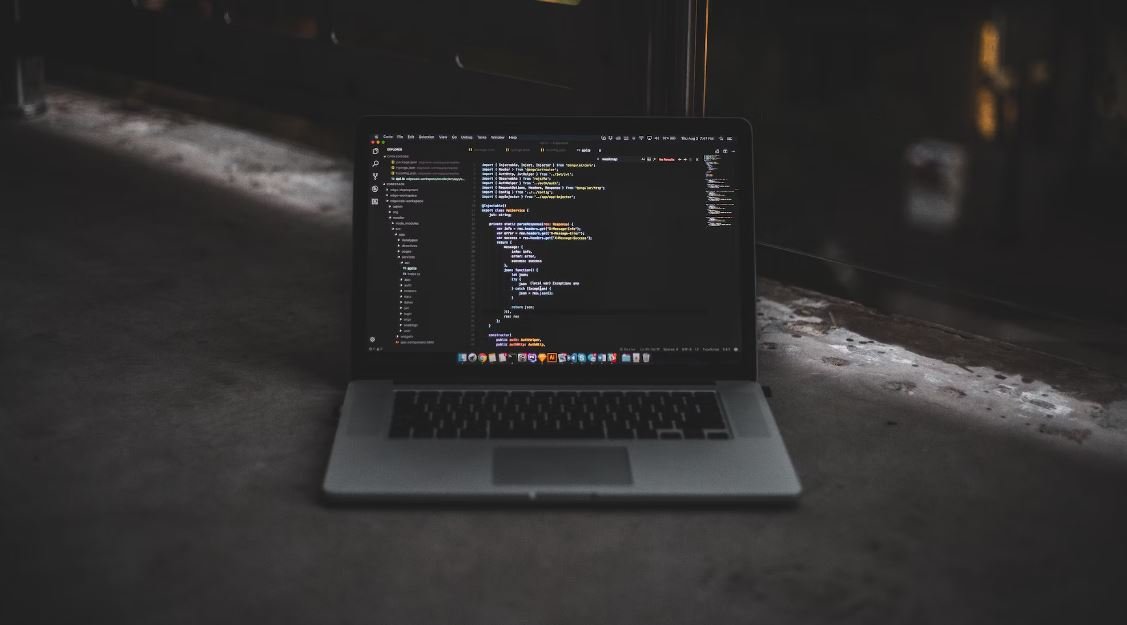
Common Misconceptions
Misconception 1: Writing XML in C# is complex
Many people believe that writing XML in C# is a complex and cumbersome process. However, this is not entirely true. While XML can be a bit tricky to understand initially, once you grasp the basic concepts, it becomes fairly straightforward to write XML in C#.
- XML documentation in C# simplifies the process of writing XML by allowing you to generate XML documentation comments automatically.
- There are various libraries available in C# that provide convenient methods and classes for working with XML, such as XElement and XDocument, which make the process easier.
- The .NET framework includes built-in support for XML serialization and deserialization, enabling you to easily convert XML data to and from C# objects.
Misconception 2: Writing XML in C# is slow
Another misconception is that writing XML in C# is slow and inefficient. While it is true that parsing and manipulating large XML files can be resource-intensive, C# provides efficient ways to handle XML processing, ensuring optimal performance.
- By using the XmlReader class instead of loading the entire XML into memory, you can efficiently process XML files of any size, without consuming excessive memory.
- Utilizing techniques such as XPath to locate and extract specific data from XML can significantly improve performance, as it allows for selective parsing.
- C# provides asynchronous programming capabilities, which enable you to perform XML operations concurrently and reduce overall processing time.
Misconception 3: Writing XML in C# is only for advanced developers
Some people think that writing XML in C# is an advanced skill that only experienced developers can handle. However, XML is a widely used markup language, and C# provides beginner-friendly tools that make it accessible to developers of all skill levels.
- Visual Studio, the popular integrated development environment for C#, includes built-in XML editing features, including auto-completion and syntax highlighting, making it easier for beginners to write XML.
- There are numerous online resources, tutorials, and documentation available that can help beginners learn the basics of XML in C#.
- C# libraries, such as LINQ to XML, provide a more intuitive and beginner-friendly way of working with XML by utilizing familiar language constructs.
Misconception 4: Writing XML in C# is limited to XML configuration files
Many people associate writing XML in C# with creating XML configuration files, such as web.config or app.config. However, XML can be used for a wide range of purposes in C#, beyond just configuration.
- XML can be used to exchange data between different systems or applications, making it a suitable format for data integration scenarios.
- Writing XML in C# allows you to create and manipulate XML documents dynamically, enabling you to generate XML for various purposes, such as generating reports or producing data feeds.
- C# provides libraries to transform XML using XSLT, giving you the ability to convert XML data into different formats, such as HTML or PDF.
Misconception 5: Writing XML in C# is prone to errors and difficult to debug
Some developers believe that writing XML in C# is error-prone and challenging to debug due to the nature of XML’s syntax and the complexity of XML-related operations. While XML can introduce some unique challenges, C# offers robust tools and techniques to handle errors and debug XML-related issues.
- The built-in XML validation capabilities in C# allow you to validate XML against a schema, helping catch errors and ensuring the XML’s validity.
- Using debugging tools in Visual Studio, you can step through your C# code and examine the XML data at runtime, making it easier to identify and fix issues.
- C# libraries, such as XMLDiffPatch, offer powerful comparison and differencing capabilities, allowing you to detect and resolve differences between XML documents during debugging.

Introduction
In this article, we will explore the process of writing XML in C#. XML (Extensible Markup Language) is a widely used language for storing and transporting data. We will provide examples and details on various aspects of XML writing in the C# programming language.
Table: XML Tags
In XML, different tags are used to define the structure and elements of a document.
Tag | Description |
---|---|
<book> | Represents a book element |
<title> | Specifies the title of the book |
<author> | Indicates the author of the book |
Table: XML Attributes
Attributes provide additional information about XML elements.
Attribute | Description |
---|---|
id | Defines a unique identifier for an element |
type | Specifies the data type of an element |
lang | Indicates the language used in the content |
Table: XML Element Types
XML supports various types of elements based on their content.
Element Type | Description |
---|---|
Empty | An element without any content |
Text | An element containing textual data |
Comment | A comment within the XML document |
Table: XML Validation
XML documents can be validated against a specified schema.
Validation Type | Description |
---|---|
Document Type Definition (DTD) | An older schema validation method |
XML Schema Definition (XSD) | A more modern and widely used schema validation method |
Relax NG | An alternative schema validation method |
Table: XML Parsing
Parsing XML involves extracting data from an XML document.
Parsing Method | Description |
---|---|
DOM (Document Object Model) | Represents the XML document as a tree structure in memory |
SAX (Simple API for XML) | Event-driven parsing, processing XML sequentially |
LINQ to XML | Provides querying and manipulating XML data in a concise way |
Table: XML Transformation
XML transformation involves converting XML documents into different formats.
Transformation Type | Description |
---|---|
XSLT (Extensible Stylesheet Language Transformations) | Defines how to transform XML using stylesheets |
XQuery | Used for querying and extracting data from XML documents |
XInclude | Enables modular inclusion of XML fragments |
Table: XML Serialization
Serialization is the process of converting XML documents into binary or text formats.
Serialization Format | Description |
---|---|
XML | The original XML format |
JSON | A popular data interchange format |
Binary | Compact representation of XML for efficiency |
Table: XML Security
XML provides mechanisms for ensuring the security of data.
Security Mechanism | Description |
---|---|
XML Encryption | Encrypts sensitive data within XML documents |
XML Signature | Verifies the authenticity and integrity of XML documents |
Access Control Lists | Defines permissions and access rights for XML resources |
Conclusion
Writing XML in C# involves understanding the different tags, attributes, element types, validation, parsing, transformation, serialization, and security mechanisms. By leveraging the power of C#, developers can effectively manipulate and process XML data in their applications. XML continues to be a vital and widely used data format, facilitating the exchange of information across various systems and platforms.
Frequently Asked Questions
Writing XML in C#
How do I write XML in C#?
To write XML in C#, you can make use of the System.Xml namespace which provides various classes and methods for XML manipulation. You can create an XmlWriter instance and use its methods to generate XML content.
What is the purpose of XMLWriter in C#?
The XMLWriter class in C# is used to produce XML data in a forward-only manner. It provides a set of methods to write XML content, allowing you to easily create well-formed XML documents or fragments.
How do I create an XML file using XMLWriter in C#?
To create an XML file using XMLWriter in C#, you can initialize an instance of XmlWriter with the desired output stream or file path. Then, you can use the WriteStartDocument, WriteStartElement, WriteEndElement, and WriteValue methods to construct the desired XML structure.
Can I write XML attributes using XMLWriter in C#?
Yes, you can write XML attributes using XMLWriter in C#. After calling the WriteStartElement method, you can use the WriteAttributeString method to add attributes to the current element.
How can I add XML namespaces using XMLWriter in C#?
To add XML namespaces using XMLWriter in C#, you can use the WriteStartNamespace and WriteEndNamespace methods. These methods allow you to define and manage the namespaces within your XML document.
What encoding options are available when writing XML in C#?
When writing XML in C#, you can specify the desired encoding by using the WriteStartDocument method overload that accepts an Encoding parameter. This allows you to choose from a variety of encodings such as UTF-8, UTF-16, ASCII, and more.
How do I handle special characters when writing XML in C#?
To handle special characters when writing XML in C#, you can use the WriteString method of XMLWriter. This ensures that special characters like ‘<', '>‘, ‘&’, and ‘”‘ are properly escaped in the generated XML content.
Can XMLWriter handle large XML files in C#?
Yes, XMLWriter can handle large XML files in C#. It uses a forward-only, non-cached approach which allows it to efficiently write XML content without requiring a large amount of memory. This makes it suitable for generating XML files of any size.
Is XMLWriter the only way to write XML in C#?
No, XMLWriter is not the only way to write XML in C#. You can also use other approaches, such as XMLDocument or XDocument classes provided by the System.Xml.Linq namespace. These classes offer more convenient and higher-level APIs for XML manipulation.
Are there any performance considerations when using XMLWriter in C#?
When using XMLWriter in C#, it is important to consider performance aspects, especially if you are dealing with large XML files or high-frequency XML generation. In such cases, it is recommended to use techniques like buffering or asynchronous writing to optimize the performance of XML generation.