Writing Unit Tests in Java
Unit testing is an essential part of software development as it helps ensure the correctness and robustness of code. In Java, there are several frameworks available for writing unit tests. This article will explore the basics of writing unit tests in Java using the popular JUnit framework.
Key Takeaways
- Unit testing is crucial for validating the correctness of code.
- JUnit is a widely-used framework for writing unit tests in Java.
- Test-driven development (TDD) is an approach in which tests are written prior to implementing the actual code.
Setting Up JUnit
To start writing unit tests in Java, you need to set up the JUnit framework in your project. First, you need to include the JUnit dependency in your project’s build configuration file, such as Maven’s pom.xml or Gradle’s build.gradle. After including the dependency, you can import the necessary classes and annotations provided by JUnit in your test files.
Setting up JUnit is straightforward and can be done easily by configuring the project’s build file.
Writing Test Methods
In JUnit, each test case is represented by a method annotated with the @Test annotation. These test methods should be declared as public and have a void return type. Inside each test method, you can utilize various assertion methods provided by JUnit to check the expected behavior of different units of your code.
Writing test methods gives developers the ability to validate the behavior of individual units of code, ensuring their correctness.
Organizing Test Cases
As your project grows, it becomes important to organize your test cases effectively. JUnit provides several annotations to group and categorize test cases. You can use the @BeforeClass and @AfterClass annotations to set up and tear down resources for an entire test class. The @Before and @After annotations allow you to perform setup and teardown operations before and after each test method execution.
Organizing test cases helps in maintainability and makes it easier to manage and update tests as the codebase evolves.
Best Practices for Writing Unit Tests
When writing unit tests in Java, it’s important to follow some best practices to ensure the effectiveness and maintainability of your test suite. Here are a few recommended practices:
- Write test cases that cover different scenarios and edge cases.
- Use meaningful names for test methods and variables to make the tests more readable.
- Avoid writing tests that are too dependent on implementation details.
- Mock or stub external dependencies to isolate the unit being tested.
- Regularly refactor and update test code as the project evolves.
Following best practices leads to more robust and reliable unit tests, enhancing the overall quality of the codebase.
JUnit and Java Versions Compatibility
JUnit versions are released in alignment with specific Java versions. Table 1 illustrates the compatibility between different versions of JUnit and Java.
JUnit Version | Java Version |
---|---|
JUnit 4 | Java 5 |
JUnit 5 | Java 8+ |
JUnit 5 requires Java 8 or higher for its usage, so if you’re working with older Java versions, JUnit 4 is the suitable option.
JUnit Annotations
Table 2 lists some of the commonly used annotations provided by JUnit.
Annotation | Description |
---|---|
@Test | Marks a method as a test method. |
@Before | Executed before each test method. |
@After | Executed after each test method. |
@BeforeClass | Executed once before any test method in the class. |
@AfterClass | Executed once after all test methods in the class have been executed. |
Using the appropriate JUnit annotations helps in organizing and controlling the execution flow of test cases.
JUnit Assertions
JUnit provides a wide range of assertion methods to validate expected results. Table 3 summarizes some commonly used assertion methods.
Method | Description |
---|---|
assertEquals() | Checks if two values are equal. |
assertTrue() | Checks if a condition is true. |
assertFalse() | Checks if a condition is false. |
assertNull() | Checks if a value is null. |
assertNotNull() | Checks if a value is not null. |
JUnit’s assertion methods play a crucial role in validating the expected behavior of your code.
Wrapping Up
In conclusion, writing unit tests in Java using the JUnit framework is an integral part of software development. By following established best practices and utilizing the various features provided by JUnit, you can ensure the correctness and reliability of your code. Writing comprehensive tests and continuously improving them as the project evolves will lead to a more stable and maintainable codebase.
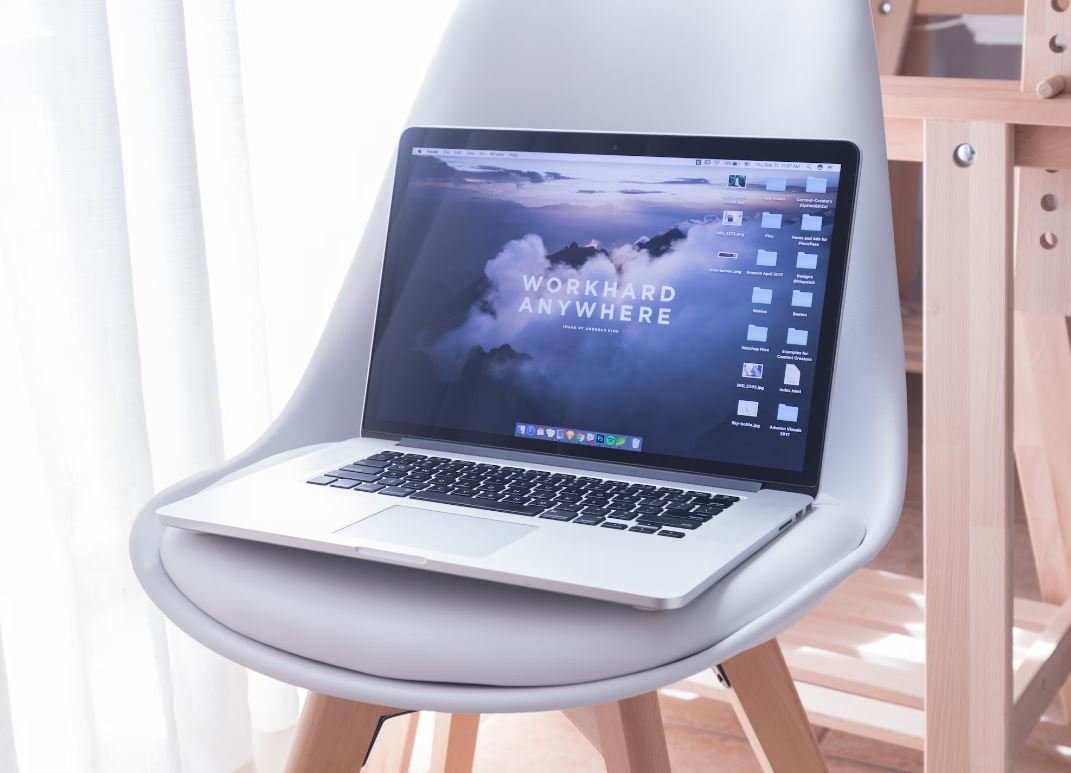
Common Misconceptions
Misconception 1: Writing unit tests is time-consuming and not worth the effort
Many developers believe that writing unit tests takes up too much time and doesn’t provide enough value compared to the time invested. However, this is a misconception because unit tests can save time in the long run by catching bugs early, improving code quality, and making it easier to refactor code.
- Unit tests can catch bugs early, preventing them from causing bigger issues later on.
- Writing tests forces you to think about the functionality of your code, leading to better design decisions.
- Unit tests act as documentation, providing insights into how the code is expected to work.
Misconception 2: Unit tests only check individual methods or functions
There is a common misconception that unit tests only focus on testing individual methods or functions in isolation, without considering the interactions between different components. However, unit tests can and should cover the interactions between components as well.
- Unit tests can verify that different components work together as expected.
- Tests can simulate interactions between components using mocking or stubbing.
- Testing interactions ensures that the overall behavior of the system is correct.
Misconception 3: Unit tests are too fragile and require constant maintenance
Some developers believe that unit tests are fragile because they need to be constantly updated whenever the code changes. While it is true that tests should be updated when the code they are testing changes, this does not mean that they are too fragile or require excessive maintenance.
- Well-designed tests with good test coverage are less likely to break with code changes.
- Unit tests are a valuable safety net when refactoring or adding new features.
- With proper setup, tests can be automatically run and integrated into the development workflow.
Misconception 4: Unit tests are not necessary if there are already integration or system tests
Some developers believe that if they already have integration or system tests in place, writing unit tests becomes unnecessary. However, unit tests serve a different purpose and complement higher-level tests.
- Unit tests provide a way to test specific behaviors in isolation, making it easier to locate and fix bugs.
- Integration or system tests may not cover all the edge cases that can be tested at the unit level.
- Unit tests can provide faster feedback during development compared to running higher-level tests.
Misconception 5: Unit testing slows down development and reduces flexibility
Another common misconception is that writing unit tests slows down the development process and makes the code less flexible. However, well-designed unit tests can actually speed up development and promote code flexibility.
- Unit tests can catch issues early on, reducing the time spent on debugging and troubleshooting.
- Tests can act as safety nets when making changes, giving confidence that the system still works as intended.
- Unit tests help to create a more maintainable codebase, which is essential for long-term flexibility.

Benefits of Unit Testing
Unit testing plays a crucial role in Java development, improving code quality and facilitating faster bug detection and debugging. Here are some of the key benefits of incorporating unit tests into your Java projects:
Benefit | Description |
---|---|
Early Bug Detection | Unit tests identify bugs early in the development process, allowing for quicker resolution. |
Improved Code Quality | Unit testing promotes cleaner code by enforcing modular design and highlighting dependencies. |
Code Refactoring | Unit tests provide a safety net for refactoring, ensuring that existing functionality is unaffected. |
Documentation of Expected Behavior | Unit tests serve as executable documentation, allowing developers to understand expected behavior. |
Unit Testing Frameworks
Different frameworks exist for writing unit tests in Java, each with its own features and capabilities. Here are a few widely used unit testing frameworks:
Framework | Description |
---|---|
JUnit | JUnit is a popular Java testing framework that provides annotations and assertions for writing tests. |
TestNG | TestNG is an alternative testing framework with more advanced features like parallel test execution and data-driven testing. |
Mockito | Mockito is a mocking framework that allows for easy creation and verification of mock objects in unit tests. |
PowerMock | PowerMock extends Mockito and other frameworks to enable mocking of static methods and final classes. |
Best Practices for Writing Unit Tests
Writing effective unit tests requires following certain best practices. Here are some guidelines:
Best Practice | Description |
---|---|
Test One Behavior per Test | Each unit test should only verify one specific behavior, making tests more focused and maintainable. |
Use Descriptive Test Names | Clear and descriptive test names improve readability and communication within the development team. |
Isolate Dependencies | Dependency isolation avoids interference between tests and ensures reliable and repeatable results. |
Test Edge Cases | Unit tests should include scenarios that test boundary conditions and exceptional cases. |
Metrics for Evaluating Test Coverage
Test coverage metrics help determine the effectiveness of unit testing. The following metrics can be used:
Metric | Description |
---|---|
Statement Coverage | Measures the percentage of code statements covered by unit tests. |
Branch Coverage | Determines the percentage of decision branches executed within unit tests. |
Path Coverage | Measures the percentage of feasible paths exercised by unit tests. |
Mutation Score | Indicates the proportion of mutated code that is detected as errors by unit tests. |
Test Doubles in Unit Testing
Test doubles, such as mocks and stubs, are essential for testing components that have external dependencies. The following types of test doubles are commonly used:
Test Double | Description |
---|---|
Mock Objects | Mocks simulate the behavior of real objects and allow for controlled verification of interactions. |
Stub Objects | Stubs provide predetermined responses to method calls, simplifying the setup of unit tests. |
Spy Objects | Spies are partial mock objects that wrap real objects, enabling monitoring and method call verification. |
Fake Objects | Fakes are simplified implementations of dependencies used in place of complex or slow components. |
Test-Driven Development (TDD)
Test-driven development (TDD) is an iterative development approach that emphasizes writing tests before writing the actual code. This technique offers several advantages:
Advantage | Description |
---|---|
Improved Design | TDD promotes modular, loosely coupled code by encouraging developers to think about the design upfront. |
Faster Feedback | By writing tests first, developers receive immediate feedback on the success or failure of their code. |
Regression Prevention | Unit tests written during TDD help prevent regressions by continuously verifying existing functionality. |
Documentation | TDD provides executable documentation that serves as an up-to-date reference for future modifications. |
Integration Testing vs. Unit Testing
Integration testing and unit testing serve different purposes in the software development lifecycle. Here are the main contrasts:
Aspect | Unit Testing | Integration Testing |
---|---|---|
Scope | Tests individual units or components in isolation. | Tests the combined behavior of multiple units or components. |
Dependencies | Mocks or stubs are used to isolate dependencies. | Real dependencies are included and may require setup. |
Granularity | Focuses on small, fine-grained functionality. | Handles larger functional units or subsystems. |
Speed | Unit tests are typically faster to execute. | Integration tests are often slower due to their broader scope. |
Unit testing in Java offers numerous benefits, such as early bug detection and code quality improvement. By following best practices, utilizing different frameworks, and exploring various test doubles, developers can build robust and maintainable Java applications. Additionally, employing test-driven development alongside integration testing allows for comprehensive software validation, reducing the risk of defects in the final product. Embracing unit testing as a fundamental component of the development process fosters software reliability and facilitates easier maintenance and future enhancements.
Frequently Asked Questions
What is a unit test?
A unit test is a type of automated test case written by a developer to ensure that a specific piece of code, usually a function or method, behaves correctly and produces the expected output.
Why are unit tests important?
Unit tests are essential in software development as they help detect bugs and ensure that code changes do not introduce new issues. They provide a safety net for code modifications, aid in identifying edge cases, and act as documentation for how the code should behave.
How do I write unit tests in Java?
To write unit tests in Java, you can utilize testing frameworks such as JUnit or TestNG. These frameworks provide annotations and assertion methods to define test cases and verify expected outcomes. You can also use mocking frameworks like Mockito to simulate dependencies.
What are some best practices for writing unit tests?
Some best practices for writing unit tests include keeping tests isolated and independent, testing both positive and negative scenarios, using descriptive test names, avoiding hardcoding test data, and regularly running tests as part of the development process.
How can I run unit tests in my Java project?
You can run unit tests in a Java project using build tools like Maven or Gradle, which have built-in support for running tests. Alternatively, most modern integrated development environments (IDEs) like IntelliJ IDEA, Eclipse, or NetBeans provide features to run tests directly from the IDE.
What is test coverage?
Test coverage refers to the extent to which your unit tests exercise your codebase. It is measured as a percentage and indicates how much of your code is tested. High test coverage helps ensure that most branches and conditions in your code are evaluated and reduces the likelihood of undiscovered defects.
How can I measure test coverage in Java?
You can measure test coverage in Java using tools like JaCoCo, which integrates with popular build tools and IDEs. These tools generate reports that show how much of your code is covered by your tests, highlighting areas that need additional testing.
Can I use unit tests to test non-public methods?
In Java, unit tests typically focus on testing public methods as they are considered the contract of a class. However, you can use reflection or other techniques to access and test non-public methods if necessary. It is recommended, though, to ensure that most of your tests are built around public API to maintain flexibility.
What is the difference between unit tests and integration tests?
Unit tests focus on testing individual components or units of code in isolation. They typically mock or stub dependencies and aim to validate the behavior of a specific unit without considering the interactions with other components. Integration tests, on the other hand, test the integration and collaboration between multiple components to ensure they work together correctly.
Are there any recommended tools or libraries for writing unit tests in Java?
Some popular tools and libraries for writing unit tests in Java include JUnit, TestNG, Mockito, and PowerMock. These frameworks provide a wide range of features to facilitate unit testing, such as annotations, assertions, and mocks. Additionally, static code analysis tools like SonarQube can help identify potential code issues that can be addressed through unit tests.