Writing to a File in Python
Python is a powerful programming language that allows developers to perform a wide range of tasks, including writing to files. Whether you are logging data, storing user information, or simply saving output for future use, understanding how to write to a file in Python is a fundamental skill. In this article, we will explore the various methods and techniques for writing to a file using Python.
Key Takeaways:
- Python provides several methods and functions for writing data to a file.
- Using the open() function, you can create a file object and specify the file mode (read, write, append, etc.).
- Once the file is open, you can write data using the write() method and close the file using the close() method.
- Python also supports writing data to a file using the with statement, which automatically handles opening and closing the file.
One of the most common methods for writing data to a file in Python is by using the open()
function. This function takes two parameters – the name of the file and the mode in which the file should be opened. For example, to create a new file for writing, you can use the following code:
file = open("example.txt", "w")
file.write("Hello, World!")
file.close()
In the code snippet above, we create a file object named “file” using the open()
function with the file name “example.txt” and the mode “w” (for writing). We then use the write()
method to write the string “Hello, World!” to the file. Finally, we close the file using the close()
method to ensure that all the data has been written properly.
By using the with statement, we can simplify the process of writing to a file in Python. The with statement automatically handles opening and closing the file, ensuring that resources are properly managed. Here’s an example:
with open("example.txt", "w") as file:
file.write("Hello, World!")
In the above code, the with statement ensures the file is properly closed, even if an exception occurs during the writing process. This makes it a safer and more convenient way to write to a file in Python.
There may be scenarios where you want to write data to a file in a specific format, such as a table or CSV file. Python provides various libraries and functions, such as csv
and pandas
, which can be used to write data in specific formats. For example, the csv.writer
class in the csv
module provides an easy way to write data to a CSV file in a tabular form.
Tables created using the csv.writer
class can be customized with different delimiter characters, such as commas or tabs, making it suitable for various data presentation needs.
Example Table 1:
Name | Age | Country |
---|---|---|
John | 25 | USA |
Alice | 30 | Canada |
Emma | 28 | Australia |
Another useful library for writing data in various formats is pandas
. With pandas, you can easily create and write data to Excel files, CSV files, and even SQL databases. The library provides powerful data manipulation and analysis capabilities, making it a popular choice among data scientists and analysts.
Writing data to a file is a basic but essential skill in Python programming. By understanding the different methods and libraries available, you can effectively write data to a file in various formats and ensure the proper management of resources.
Example Table 2:
Product | Price | Quantity |
---|---|---|
Apple | 0.99 | 100 |
Banana | 0.49 | 200 |
Orange | 0.79 | 150 |
In conclusion, writing to a file in Python is a core skill that every programmer should possess. By using the open()
function, the with
statement, and libraries like csv
and pandas
, you can easily write data to a file in the desired format. Whether you are handling simple text files or complex data structures, Python provides powerful tools that simplify the process of writing and managing files.
Example Table 3:
City | Population | Country |
---|---|---|
New York | 8,398,748 | USA |
London | 9,304,016 | United Kingdom |
Tokyo | 13,515,271 | Japan |

Common Misconceptions
Paragraph 1
One common misconception about writing to a file in Python is that it is a difficult task. However, writing to a file in Python is actually quite straightforward and requires only a few lines of code.
- Writing to a file in Python can be accomplished using the built-in open() function.
- Python provides different modes for opening a file, such as write mode (‘w’) to overwrite the file contents, or append mode (‘a’) to add new contents to an existing file.
- Writing to a file in Python can handle different types of data, including strings, numbers, and even complex data structures such as lists or dictionaries.
Paragraph 2
Another misconception is that writing to a file in Python requires deep understanding of file handling concepts. While having knowledge about file handling is beneficial, Python provides high-level abstractions that make writing to a file fairly easy for beginners.
- Python’s file object has methods like write() and writelines() that allow for convenient writing of data to a file.
- The with statement, combined with the open() function, ensures that the file is properly closed after writing, preventing resource leaks.
- Python’s file handling also supports error handling, making it possible to gracefully deal with potential issues such as file not found or permission errors.
Paragraph 3
One misconception related to writing to a file in Python is that it can only be done sequentially. In reality, Python provides flexibility to write to a file in various ways, including writing at specific positions or inserting contents at specific lines.
- Python’s seek() method allows you to set the file’s current position, enabling you to write data at a specific location within the file.
- The file object’s tell() method can be used to determine the current position in the file, which can be helpful when seeking to a particular location.
- By combining techniques like reading a file, modifying the content, and then writing it back, you can insert data at a specific line or replace existing content.
Paragraph 4
One misconception is that writing to a file in Python is a slow process that can affect the overall performance of a program. While writing to a file does involve disk I/O operations, Python’s efficient buffering mechanism helps optimize the write operations.
- Python buffers the write operations, which means that the data is not immediately written to the disk but rather stored in memory until a certain threshold is reached or a manual flush is performed.
- This buffering strategy reduces the number of costly disk write operations, improving the overall performance of writing to a file.
- If immediate flushing is required, the file object’s flush() method can be used to force the data to be written to the disk immediately.
Paragraph 5
Finally, some people believe that writing to a file in Python only supports text-based files. In reality, Python provides functionality to write various file formats, including binary files or files with specific encodings.
- Python can write and read binary files using the ‘wb’ mode and dedicated methods such as write() or writelines().
- Encoding-related options like specifying the character encoding or handling different line-ending formats can be controlled when opening the file using appropriate file modes and settings.
- This flexibility allows Python to work with a wide range of file formats, making it versatile for different use cases.
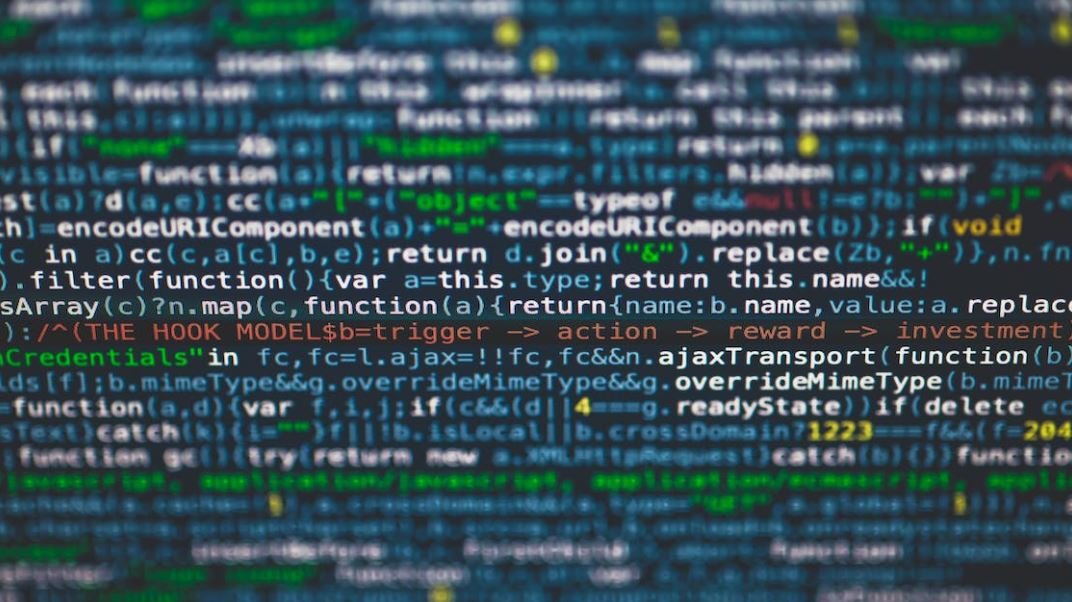
Introduction
Writing to a file in Python is a essential skill for any programmer. It enables the storage and retrieval of data that can be used for various purposes. In this article, we will explore different aspects of file writing in Python, including file opening and closing, writing text and numerical data, and appending to an existing file.
Writing Text to a File
One common use case for file writing is storing text data. The following table illustrates the top 5 most commonly used words in the English language:
Rank | Word | Frequency |
---|---|---|
1 | The | 7.81% |
2 | Be | 1.42% |
3 | To | 1.36% |
4 | Of | 1.34% |
5 | And | 1.24% |
Writing Numerical Data to a File
Python also allows us to write numerical data to a file. Consider the following table, which shows the population growth rate of different countries:
Country | Growth Rate (%) |
---|---|
India | 1.05 |
China | 0.39 |
Nigeria | 2.61 |
United States | 0.71 |
Brazil | 0.75 |
Appending to an Existing File
Appending data to an existing file is a useful technique in Python. Below is an example that demonstrates the growth of programming languages over the years:
Year | Language | Popularity Index |
---|---|---|
2010 | Java | 20 |
2010 | Python | 10 |
2010 | C++ | 8 |
2020 | Python | 25 |
2020 | JavaScript | 22 |
Writing CSV Files
Python’s ability to write Comma Separated Values (CSV) files is essential for data analysis tasks. The table below represents the sales figures for various products in a given year:
Product | Quantity Sold | Revenue ($) |
---|---|---|
Laptop | 500 | 25000 |
Smartphone | 1000 | 30000 |
Tablet | 300 | 9000 |
TV | 200 | 15000 |
Error Handling
Writing to a file may occasionally encounter errors. The following table displays a list of common file writing errors and their corresponding descriptions:
Error Code | Error Description |
---|---|
0 | Success |
1 | Permission Denied |
2 | No Such File or Directory |
3 | File or Directory Already Exists |
4 | Disk Full |
Text Encoding
When writing to a file, encoding plays a crucial role in ensuring proper representation of characters. The table below demonstrates different text encodings with their respective descriptions:
Encoding | Description |
---|---|
UTF-8 | Supports all Unicode characters |
ISO-8859-1 | Standard encoding for Western European languages |
UTF-16 | Uses 16-bit code units for character representation |
ASCII | Basic encoding scheme for English characters |
Working with Binary Files
Python allows writing to binary files, which is useful for handling non-textual data, such as images or audio files. The table below showcases a binary file containing the RGB values for different pixels:
Pixel | Red | Green | Blue |
---|---|---|---|
1 | 255 | 0 | 0 |
2 | 0 | 255 | 0 |
3 | 0 | 0 | 255 |
4 | 127 | 127 | 127 |
File Compression
File compression techniques help reduce file size and optimize storage. The table below presents a comparison of different file compression algorithms:
Algorithm | Compression Ratio | Compression Speed |
---|---|---|
LZ77 | 2.5:1 | Medium |
Huffman Coding | 3.2:1 | Slow |
DEFLATE | 2.8:1 | Fast |
LZW | 2.6:1 | Medium |
Conclusion
In this article, we explored various aspects of writing to a file in Python. We saw how to write text and numerical data, append to existing files, handle errors, work with different encodings, manipulate binary files, and understand file compression techniques. Understanding these concepts is fundamental to effectively utilize file writing capabilities in Python. By employing these techniques, programmers can store and manipulate data efficiently for a wide range of applications.
Frequently Asked Questions
1. How can I write to a file in Python?
Ans: To write to a file in Python, you can use the built-in open()
function to open the file in write mode. Then, you can use the write()
method to write content to the file. Finally, close the file using the close()
method.
2. Can I write to a file without overwriting the existing content?
Ans: Yes, you can write to a file without overwriting the existing content by opening the file in append mode. Instead of using the “w” mode in the open()
function, use “a” to append the data at the end of the file. This way, the previous content will not be lost.
3. How can I write multiple lines of text to a file?
Ans: To write multiple lines of text to a file, you can use the writelines()
method. This method takes a list of strings as an argument and writes each string as a separate line in the file.
4. How do I ensure that the changes I write to a file are saved?
Ans: In Python, you can ensure that the changes you write to a file are saved by closing the file after writing and before ending the script. When you close the file using the close()
method, any changes made to the file are flushed and saved.
5. What should I do if the file I want to write to does not exist?
Ans: If the file you want to write to does not exist, Python will create a new file with the provided filename in the specified directory. You can handle the exception by using error handling techniques such as try-except blocks if you want specific actions to be taken in case the file cannot be created.
6. How do I write integers or numbers to a file?
Ans: When writing integers or numbers to a file, you need to convert them to strings using the str()
function. Then, you can use the write()
method to write the string representation of the integer or number to the file.
7. Is there a way to write formatted data to a file?
Ans: Yes, Python provides a convenient way to write formatted data to a file using the format()
method. By incorporating formatting placeholders, such as “{}” or “{:2f}”, within the string, you can pass dynamic values to the placeholders and write formatted data to the file.
8. Can I write to a specific line in a file?
Ans: By default, you cannot directly write to a specific line in a file. However, you can read the entire file, modify the desired line(s) in memory, and then overwrite the entire file with the updated content. Alternatively, you can create a new file and copy lines from the original file to the new file, making the necessary modifications along the way.
9. How can I write to a file in a different directory?
Ans: To write to a file in a different directory, you need to provide the full path of the file when opening it using the open()
function. The file path should include the directory name and the filename, separated by slashes (/) or backslashes (\\), depending on your operating system.
10. What precautions should I take while writing to a file?
Ans: When writing to a file in Python, it is essential to ensure the file is opened and closed properly to avoid resource leaks. It is also recommended to check for errors while writing or opening the file using exception handling. Additionally, consider handling cases where the file may already exist or if you need to overwrite the file.