Writing Bash Scripts
Bash scripting is a powerful skill for developers and system administrators alike. With the ability to automate tasks and execute complex commands, writing bash scripts can greatly improve efficiency and productivity. Whether you’re a beginner or an experienced user, learning to write bash scripts can enhance your workflow and simplify repetitive tasks.
Key Takeaways
- Bash scripting allows for task automation and efficient execution of commands.
- Learning to write bash scripts can benefit beginners and experienced users.
- Bash scripts improve workflow and simplify repetitive tasks.
Getting Started with Bash Scripts
Before diving into bash scripting, it’s important to understand the basics. Bash, short for “Bourne Again SHell,” is a command-line language used to interact with operating systems. It is the default shell on most Linux distributions and macOS, offering a wide range of available commands. To start writing bash scripts, all you need is a text editor.
With just a text editor, you can unleash the power of the command line.
The Structure of a Bash Script
A bash script follows a specific structure to ensure proper execution. Here is a simple breakdown:
- Shebang: Begins with
#!/bin/bash
and specifies the interpreter. - Comments: Optional lines starting with
#
to add documentation and clarify script functionality. - Commands: The core of the script, containing the instructions and operations to be executed.
- Variables: Optional variables can be defined to store and manipulate data.
- Functions: Reusable blocks of code that help organize and modularize the script.
By following this structure, your bash scripts will be well-organized and easily maintainable.
Useful Tips and Techniques
When writing bash scripts, consider the following helpful tips and techniques:
- Use proper indentation and formatting for better readability.
- Double-quote variables to handle spaces and special characters correctly.
- Redirect and capture command output using
>
,>>
, and&>
. - Employ conditional statements and loops for decision-making and repetitive tasks.
Utilizing these tips will greatly enhance the quality and functionality of your bash scripts.
Tables
Scripting Concepts | Description |
---|---|
Variables | Used to store and manipulate data within the script. |
Functions | Reusable blocks of code that perform specific tasks. |
Useful Commands | Description |
---|---|
echo | Prints text or variables to the standard output. |
if | Executes commands based on a specified condition. |
for | Loops over a sequence of values or elements. |
Best Practices | Description |
---|---|
Modularity | Break down the script into functions for better organization. |
Error Handling | Include proper error handling to prevent unexpected behavior. |
Advanced Bash Scripting
To become an expert in bash scripting, consider exploring the more advanced topics:
- Regular expressions: Pattern matching and text manipulation.
- Command-line arguments: Parameters passed to a script during execution.
- File handling: Reading, writing, and manipulating files.
Exploring advanced topics will expand your bash scripting capabilities and empower you to handle complex scenarios.
Wrapping It Up
Writing bash scripts is a valuable skill that can greatly improve productivity. By automating tasks and executing complex commands with ease, developers and system administrators can save time and effort. Whether you’re a beginner or looking to enhance your existing skills, learning bash scripting will prove beneficial for your daily workflow.
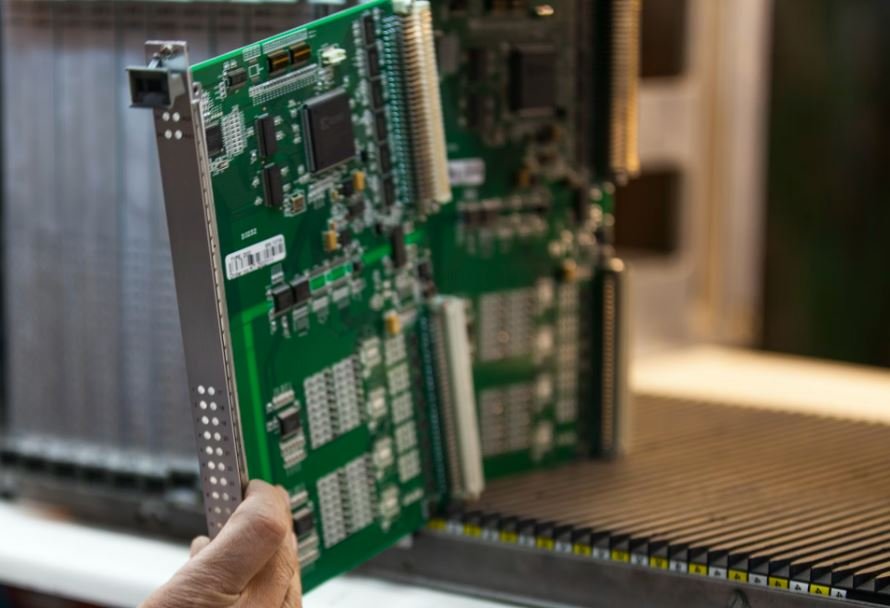
Common Misconceptions
Misconception 1: Bash scripting is only for advanced programmers
One common misconception about writing Bash scripts is that it is a skill reserved only for advanced programmers. However, this is not true. Bash scripting can be learned by anyone, regardless of their programming experience. It is a powerful tool that can help automate repetitive tasks and simplify complex tasks. With some basic knowledge and practice, even beginners can start writing Bash scripts.
- Bash scripting can be learned by beginners
- No prior programming experience is required
- Basic knowledge is sufficient to start writing Bash scripts
Misconception 2: Bash scripts are only useful in Linux environments
Another misconception is that Bash scripts are only useful in Linux environments. While it is true that Bash is the default shell in most Linux distributions, Bash scripts can also be run on other operating systems, including macOS and Windows (using tools like Git Bash). This cross-platform compatibility makes Bash scripts versatile and widely applicable. It allows users to automate tasks on different systems, not just limited to Linux.
- Bash scripts can be run on macOS and Windows too
- Bash is not exclusive to Linux
- Allows scripting on different operating systems
Misconception 3: Bash scripting is slow and inefficient
One of the common misconceptions about Bash scripting is that it is slow and inefficient compared to other programming languages. While it is true that Bash scripts may not be as efficient as compiled languages like C or Java, they are generally fast enough for most tasks. Bash scripts are designed to automate system administration tasks and process text, where performance is often not a critical factor. Moreover, Bash scripts can call external programs, allowing users to leverage the speed and efficiency of other languages when needed.
- Bash scripts are generally fast enough for most tasks
- Performance is not always critical in system administration tasks
- Bash scripts can leverage the speed of other languages when needed
Misconception 4: Bash scripting is only suitable for simple tasks
Some people believe that Bash scripting is only suitable for simple tasks and cannot handle complex tasks. However, this is not true. Bash has a wide range of features and supports various programming constructs, such as conditionals, loops, functions, and variables, which allow for the creation of complex scripts. With the right knowledge and practice, Bash scripts can handle a variety of tasks, from simple one-liners to large-scale automation projects.
- Bash supports conditionals, loops, functions, and variables
- Bash scripting is not limited to simple tasks
- Complex scripts can be created with Bash
Misconception 5: Bash scripting is insecure and prone to vulnerabilities
There is a misconception that Bash scripting is insecure and prone to vulnerabilities. While it is true that writing insecure code can lead to vulnerabilities, this is not unique to Bash scripting. As with any programming language, it is important to follow best practices and ensure proper input validation, error handling, and security measures when writing Bash scripts. By adhering to these practices, Bash scripts can be written securely and without exposing systems to unnecessary risks.
- Security vulnerabilities can be mitigated by following best practices
- Proper input validation and error handling are important in Bash scripts
- Bash scripts can be written securely by implementing security measures
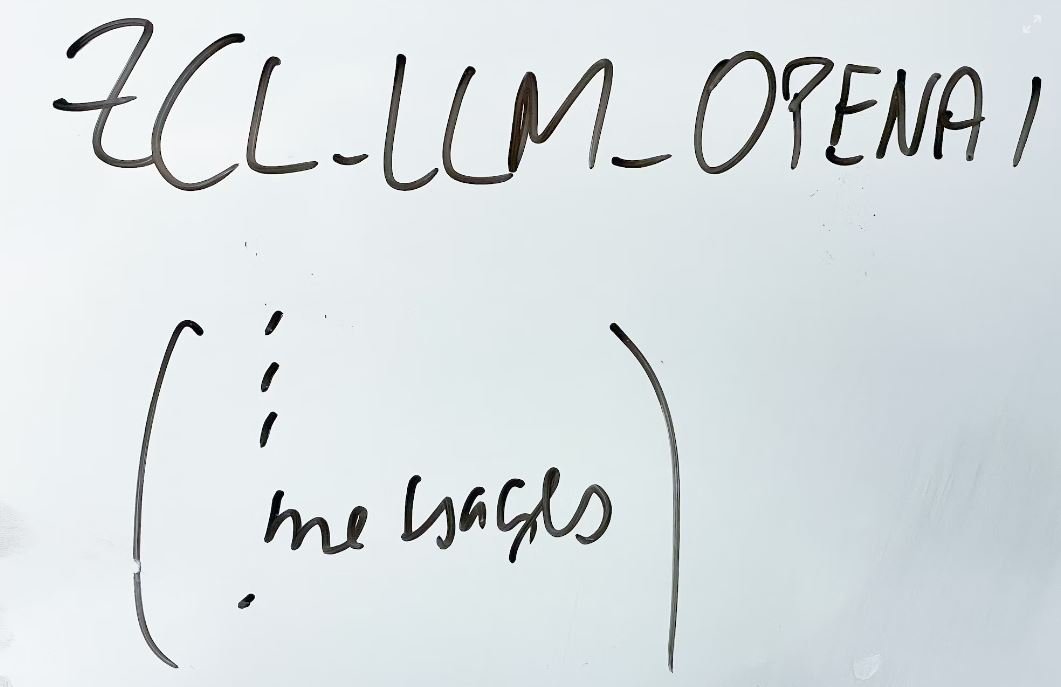
Importance of Bash Scripts
Bash scripting is an essential skill for developers and system administrators to automate repetitive tasks, improve productivity, and ensure consistency in their work. Below are ten interesting examples showcasing various applications and benefits of writing Bash scripts.
1. Files and Directories
In a directory containing 100 files and 20 subdirectories, a Bash script to count the number of files and directories could be:
Command | Output |
---|---|
ls -p | grep -v / | wc -l |
100 |
ls -p | grep / | wc -l |
20 |
2. System Monitoring
Bash scripts can be used to monitor system resources, like CPU usage, memory consumption, and disk space utilization. The following script calculates the CPU usage percentage:
Command | Output |
---|---|
top -b -n 1 | grep "Cpu(s)" | awk '{print $2 + $4}' |
14.5% |
3. Network Services
Automating the management of network services is another powerful use case. The example below restarts the Apache web server:
Command | Output |
---|---|
systemctl restart apache2 |
Server successfully restarted |
4. Database Operations
Bash scripts can streamline database administration tasks, such as creating backups. The script below creates a backup of MySQL databases:
Command | Output |
---|---|
mysqldump -u root -p password --all-databases > backup.sql |
Backup created successfully |
5. Text Processing
Bash scripts excel at manipulating and processing text efficiently. The following script extracts email addresses from a text file:
Command | Output |
---|---|
grep -E -o "[[:alnum:]_.-]+@[[:alnum:]_-]+\.[[:alpha:].]+" file.txt
|
example@example.com test@test.com |
6. File Compression
Bash scripts can automate the compression of files or directories. This script compresses a directory using tar and gzip:
Command | Output |
---|---|
tar -czf archive.tar.gz directory
|
Archive created successfully |
7. File Permissions
Managing file permissions is crucial for security. The script below changes file permissions recursively:
Command | Output |
---|---|
chmod -R 755 directory |
Permissions changed successfully |
8. File Transfer
Transferring files between systems can be automated using Bash scripts. Here’s an example using Secure Copy:
Command | Output |
---|---|
scp file.txt user@remote:/path/to/destination |
File successfully transferred |
9. Error Handling
Bash scripts provide mechanisms for handling errors gracefully. The script below checks if a file exists and displays a custom message:
Command | Output |
---|---|
if [ -f file.txt ]; then echo "File exists"; else echo "File does not exist"; fi
|
File exists |
10. Cron Jobs
Bash scripts are commonly used with cron to schedule automated tasks. The example below executes a script every hour:
Command | Output |
---|---|
0 * * * * /path/to/script.sh |
Script executed |
From managing files and directories to automating system tasks, Bash scripts offer an array of functionality that saves time and enhances productivity. Mastering this scripting language equips developers and administrators with an invaluable skillset, enabling them to efficiently solve complex problems and optimize their workflows.
Frequently Asked Questions
What is Bash?
Bash (Bourne Again SHell) is a command-line interpreter and scripting language used in Unix-like operating systems. It is the default shell in most Linux distributions and macOS.
How do I write a Bash script?
To write a Bash script, create a new file with a .sh extension and start each line with a Bash command or script. Make the file executable using the chmod command, and then you can run the script using the ./filename.sh command in the terminal.
What are the advantages of writing Bash scripts?
Writing Bash scripts can automate repetitive tasks, enhance productivity, and save time. It allows you to combine different commands and create complex workflows. Bash scripts are portable and can be run on any system with Bash installed.
How can I debug a Bash script?
You can debug a Bash script by using the set -x command to enable debug mode, which displays each command before executing it. You can also use the echo command to print variable values and debug statements at different points in the script.
Can I run a Bash script on Windows?
While Bash is not natively available on Windows, you can install a Bash environment using tools like Git Bash or Windows Subsystem for Linux (WSL). These tools provide a command-line interface that supports executing Bash scripts on a Windows machine.
How can I pass arguments to a Bash script?
You can pass arguments to a Bash script by using command-line parameters. These arguments can be accessed within the script using special variables like $1, $2, $3, and so on. $0 represents the script name itself. You can also use options and flags to provide specific inputs.
Can I schedule a Bash script to run automatically?
Yes, you can schedule a Bash script to run automatically using tools like cron or systemd on Unix-like systems. These tools allow you to define the desired schedule and execute the script accordingly. In the script, ensure the necessary environment variables and paths are set correctly.
How can I handle errors or exceptions in a Bash script?
In Bash, you can handle errors or exceptions by using conditional statements, such as if-else or case statements, to check different conditions and handle specific scenarios. Additionally, you can use the trap command to catch and handle signals or errors that occur during script execution.
What are some best practices for writing Bash scripts?
Some best practices for writing Bash scripts include using descriptive variable names, commenting your code to improve readability, handling edge cases and errors, using indentation for better structure, and testing scripts thoroughly before deployment. It is also recommended to follow the “Don’t Repeat Yourself” (DRY) principle and modularize code for reusability.
Where can I find additional resources to learn Bash scripting?
There are several online resources available to learn Bash scripting, including tutorials, documentation, and forums. Some popular websites include Bash Academy, GNU Bash manual, Stack Overflow, and GitHub repositories with Bash script examples. You can also explore books and online courses dedicated to learning Bash scripting.