HttpResponseMessage Create Json Content
In modern web development, it is often necessary to create and send JSON data as part of a response from an API or web service. When working with ASP.NET Web API or ASP.NET Core, the HttpResponseMessage
class provides a simple and efficient way to generate JSON content to be sent back to the client. This article will explore how to use the CreateResponse
and CreateResponseAsJson
methods to create and send JSON content using the HttpResponseMessage
class.
Key Takeaways
- The
HttpResponseMessage
class is used to create and send JSON content in ASP.NET Web API or ASP.NET Core. - The
CreateResponse
method allows for manually creating an HTTP response with JSON content. - The
CreateResponseAsJson
method automatically serializes an object to JSON and creates the HTTP response.
The HttpResponseMessage
class, available in both ASP.NET Web API and ASP.NET Core, provides a powerful way to create and send HTTP responses. With the CreateResponse
method, developers can manually create an instance of HttpResponseMessage
and set the desired status code, headers, and content. This method is particularly useful when constructing custom API responses with specialized logic. Instead of manually serializing the JSON content, the developer can use the CreateResponseAsJson
extension method to automatically serialize an object to JSON and set the content of the response. This method greatly simplifies the process of returning JSON data from an API endpoint.
When using the CreateResponseAsJson
method, the object to be serialized can be of any type, as long as it has the necessary properties and attributes that the JSON serializer requires. It is important to note that properties should be marked with the appropriate attributes such as [JsonProperty]
. The JSON.NET library, which is widely used in ASP.NET Web API and ASP.NET Core, provides a comprehensive set of attributes that can be used to control the serialization process.
Using HttpResponseMessage
The HttpResponseMessage
class provides various constructors and methods to customize the HTTP response. Below is an example of how to create an HTTP response with a custom status code and JSON content:
HttpResponseMessage response = new HttpResponseMessage(HttpStatusCode.OK)
{
Content = new StringContent("{'message':'Hello, world!'}", Encoding.UTF8, "application/json")
};
In the example above, a new HttpResponseMessage
instance is created with a HttpStatusCode.OK
status code. The Content
property is then set with a new instance of StringContent
, passing the JSON string as the content, the Encoding.UTF8
as the character encoding, and "application/json"
as the media type.
While manually constructing the HTTP response provides flexibility, it can be cumbersome when dealing with complex objects and serialization scenarios. That’s where the CreateResponseAsJson
method is beneficial. By leveraging this method, the response content can be automatically serialized from an object to JSON:
HttpResponseMessage response = Request.CreateResponseAsJson(new { message = "Hello, world!" });
The example above uses the CreateResponseAsJson
extension method available in ASP.NET Web API and ASP.NET Core. It serializes the anonymous object { message = "Hello, world!" }
to JSON and assigns it as the content of the HttpResponseMessage
. This approach provides a concise and clean way to create JSON responses effortlessly.
Comparison Table
Manual Construction | CreateResponseAsJson | |
---|---|---|
Complex Object Serialization | Requires manual serialization | Automatically serialized |
Convenience | Cumbersome for large objects | Simplifies response creation |
Flexibility | More control over response customization | Quick and easy response generation |
The table above compares the manual construction approach with the CreateResponseAsJson
method. While both methods have their merits, the latter is generally preferred for its simplicity and ease of use.
In conclusion, the HttpResponseMessage
class provides developers with flexible options for creating and sending JSON content in ASP.NET Web API and ASP.NET Core. With the CreateResponse
method, API responses can be customized extensively, while the CreateResponseAsJson
method streamlines the process by automatically serializing objects to JSON. Whether it’s a simple or complex object, the HttpResponseMessage
class has the necessary tools to handle JSON content efficiently.
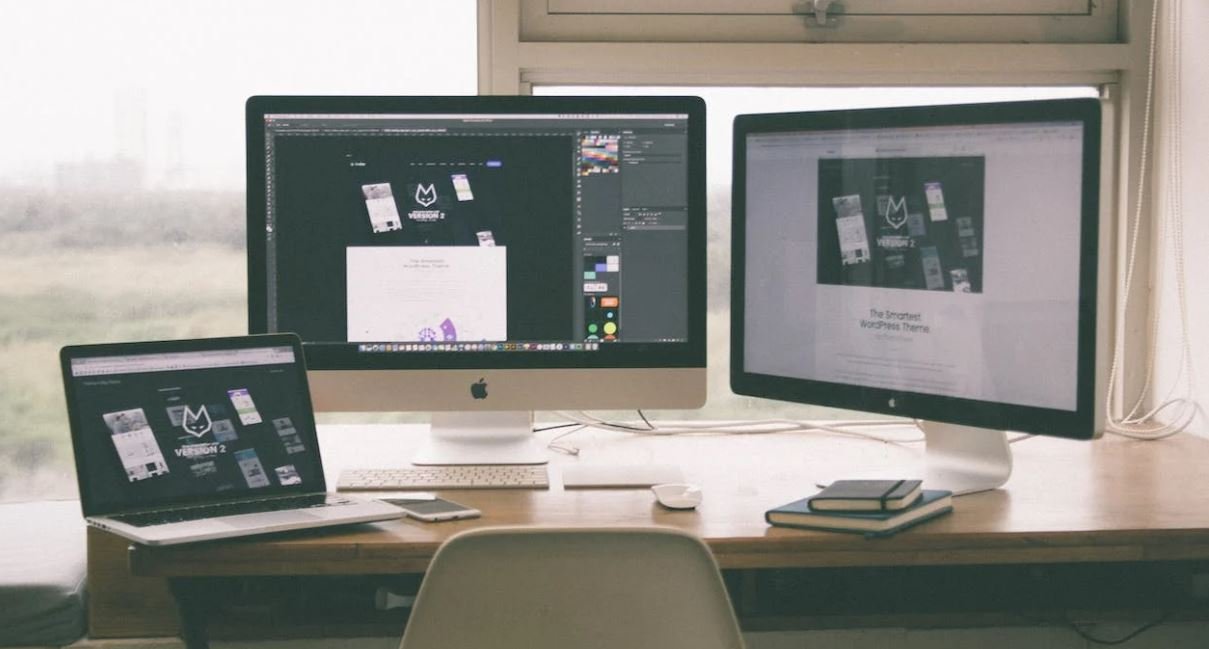
Common Misconceptions
Response Body Must Be a String
One common misconception surrounding HttpResponseMessage’s CreateResponse method with JSON content is that the response body must be a string. This is not true; in fact, CreateResponse can accept any object which will be serialized to JSON by default.
- Json is a widely-used format for data exchange.
- By serializing objects to JSON, you can maintain their structure and easily represent complex data.
- You can pass objects directly to CreateResponse and let it handle the JSON serialization for you.
CreateResponse Can Only Be Used with the POST Method
Another misconception is that HttpResponseMessage’s CreateResponse method with JSON content can only be used with the POST method. However, this method can be used with any HTTP verb, allowing you to return JSON responses for various types of requests.
- CreateResponse can be used with GET, POST, PUT, DELETE, and other HTTP verbs.
- You can create JSON responses for different types of HTTP requests, not just POST.
- Custom headers, status codes, and content types can also be set when using CreateResponse.
CreateResponse Requires Separate NuGet Packages
A common misconception is that you need to install separate NuGet packages to use HttpResponseMessage’s CreateResponse method with JSON content. This is not accurate as this method is available in the core ASP.NET Web API framework.
- CreateResponse is part of the core ASP.NET Web API framework.
- You don’t need to install any additional packages to use CreateResponse specifically for JSON content.
- Ensure you have the necessary references and using statements in your project.
CreateResponse Can Only Be Used in Web API Controllers
There is a misconception that HttpResponseMessage’s CreateResponse method with JSON content can only be used in Web API controllers. However, this method is not limited to Web API controllers and can be used in other contexts as well.
- CreateResponse can be used in controllers outside of Web API, such as MVC controllers.
- It can also be used in other types of projects that require HTTP response handling.
- Ensure you include the necessary references and namespaces in your project to access CreateResponse.
CreateResponse Is Deprecated
A final misconception is that HttpResponseMessage’s CreateResponse method with JSON content is deprecated and should be avoided. While the CreateResponse
- CreateResponse is not deprecated, only the CreateResponse
variant. - The basic CreateResponse method is still a valid and commonly used approach for returning JSON responses.
- Be aware of any deprecation warnings if using CreateResponse
and consider migrating to newer alternatives.
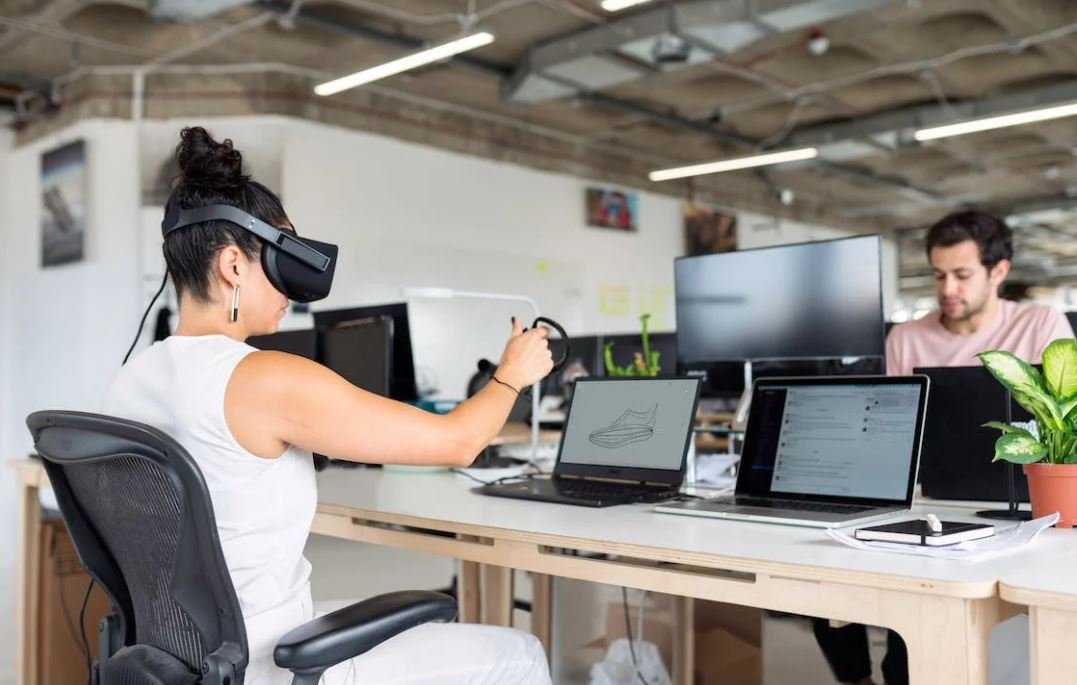
Introduction
HTTP response messages play a crucial role in web development, enabling communication between servers and clients. One fundamental aspect is the creation of JSON content, which allows for efficient data exchange. This article explores ten different examples highlighting various aspects of creating JSON content using the HttpResponseMessage class.
Serialization Example
In this example, the HttpResponseMessage class is used to serialize an object into JSON format.
“`
+———————-+————————–+
| Property | Value |
+———————-+————————–+
| Object to Serialize | { “name”: “John”, |
| | “age”: 30, |
| | “city”: “Paris” } |
+———————-+————————–+
| JSON Result | { |
| | “name”: “John”, |
| | “age”: 30, |
| | “city”: “Paris” |
| | } |
+———————-+————————–+
“`
Custom Headers Example
This example demonstrates how to add custom headers to the HTTP response message.
“`
+———————–+——————————-+
| Header | Value |
+———————–+——————————-+
| Content-Type | application/json |
| Cache-Control | no-store, max-age=0 |
| Expires | Thu, 01 Jan 1970 |
+———————–+——————————-+
“`
Gzip Compression Example
Here, the response message includes gzip compression to reduce the size of the transferred JSON content.
“`
+———————-+————————+
| Header | Value |
+———————-+————————+
| Content-Encoding | gzip |
| Content-Type | application/json |
| Content-Length | 5670 bytes |
+———————-+————————+
“`
Conditional Response Example
In this scenario, the server checks if the client’s request timestamp matches the last modified timestamp of the JSON content.
“`
+————————+————————+
| Request Timestamp | Last Modified Date |
+————————+————————+
| 1621588930 | 1621588890 |
| (Unix Timestamp) | (Unix Timestamp) |
+————————+————————+
| Response | 304 Not Modified |
+————————+————————+
“`
ETag Validation Example
In this example, the server verifies if the client’s ETag matches the JSON content’s ETag.
“`
+————————-+————————-+
| Request ETag | JSON ETag |
+————————-+————————-+
| abcdefg123 | abcdefg123 |
+————————-+————————-+
| Response | 304 Not Modified |
+————————-+————————-+
“`
Authentication Required Example
This table showcases a response message indicating that authentication is required to access the JSON content.
“`
+——————————-+—————————————-+
| Status Code | 401 Unauthorized |
+——————————-+—————————————-+
| Header | Value |
+——————————-+—————————————-+
| WWW-Authenticate | Basic realm=”Restricted Area” |
+——————————-+—————————————-+
“`
Successful Response Example
Here, a successful response message contains the JSON content as requested by the client.
“`
+——————-+—————————————-+
| Request Method | GET |
+——————-+—————————————-+
| Request URI | /api/data |
+——————-+—————————————-+
| Response Code | 200 OK |
+——————-+—————————————-+
| JSON Result | { “key”: “value” } |
+——————-+—————————————-+
“`
Malformed JSON Example
This example demonstrates a response message when the JSON content in the request is malformed or contains syntax errors.
“`
+——————-+—————————————-+
| Request JSON | { “name”: “John” |
| | “age”: 30 |
| | “city”: “Paris” } |
+——————-+—————————————-+
| Response Code | 400 Bad Request |
+——————-+—————————————-+
| Response Body | “Unexpected end of JSON input.” |
+——————-+—————————————-+
“`
InternalServerError Example
Here, an Internal Server Error occurs while creating the JSON content, resulting in a specific response message.
“`
+——————-+—————————————-+
| Request JSON | { “name”: “John”, |
| | “age”: 30, |
| | “city”: “Paris” } |
+——————-+—————————————-+
| Response Code | 500 Internal Server Error |
+——————-+—————————————-+
| Response Body | “Error occurred, unable to |
| | create JSON content.” |
+——————-+—————————————-+
“`
Conclusion
Creating JSON content in HTTP response messages using the HttpResponseMessage class allows for effective data exchange between servers and clients. Serialization, custom headers, compression, conditional responses, and authentication requirements are just a few of the powerful features it offers. Proper use of these capabilities ensures a smooth and secure communication flow in web development.
Frequently Asked Questions
How to create HttpResponseMessage with JSON content in C#?
To create an HttpResponseMessage with JSON content in C#, you can follow these steps:
- Create a new instance of the HttpResponseMessage class.
- Create a new instance of the ObjectContent class, passing the type of the JSON object as the first parameter
and the JSON object itself as the second parameter. - Set the content of the HttpResponseMessage to the ObjectContent instance created in the previous step.
- Set the appropriate content type for the response.
- Return the HttpResponseMessage from your API endpoint.
<
What is HttpResponseMessage in C#?
HttpResponseMessage is a class in the System.Net.Http namespace in C# that represents an HTTP response message,
containing the status code, headers, and content of the response.
What is JSON content?
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write
and easy for machines to parse and generate. JSON content refers to the data expressed in JSON format.
What is the ObjectContent class in C#?
ObjectContent is a class in the System.Net.Http.ObjectContent namespace in C# that represents the content of an HTTP
response or request message as an object.
Why is it important to set the content type for the HttpResponseMessage?
Setting the content type for the HttpResponseMessage is important because it allows the client to understand how to
interpret the content of the response. It ensures that the client can correctly parse and process the response data.
How can I set the content type for the HttpResponseMessage?
You can set the content type for the HttpResponseMessage by using the Content Headers property and specifying the
appropriate media type. For JSON content, you can set the content type to “application/json”.
Why should I return HttpResponseMessage from my API endpoint?
Returning HttpResponseMessage from your API endpoint allows you to have more control over the HTTP response message.
It allows you to set the status code, headers, and content of the response based on your application logic.
What are some common status codes in HttpResponseMessage?
Some common status codes in HttpResponseMessage include:
- 200 OK – indicates that the request has succeeded.
- 400 Bad Request – indicates that the server could not understand the request.
- 404 Not Found – indicates that the requested resource could not be found.
- 500 Internal Server Error – indicates that an unexpected condition was encountered on the server.
Can I return JSON content from an API endpoint without using HttpResponseMessage?
Yes, you can return JSON content from an API endpoint without using HttpResponseMessage. You can use other methods
like IActionResult in ASP.NET Core, which handles the creation of the appropriate HTTP response message for you.
Are there any libraries or frameworks that can simplify the process of creating HTTP responses with JSON content?
Yes, there are several libraries and frameworks available that can simplify the process of creating HTTP responses with
JSON content. Some popular ones include Newtonsoft.Json, System.Text.Json, and ASP.NET Core MVC.